
TYPEWRITER TEXT EFFECT
|
Note: As of today (Dec 2003), this code works on Internet Explorer
6 and Netscape 6.2 and 7.02 on the PC. It has not been tested on other browsers.
|
EXAMPLE
|
You can type some text here and click
the button below to see the effect applied to the new text.
Delay:
milliseconds
between strokes.
|
EXPLANATION
|
As you can see
from the example, this effect shows a sequences of characters letter by
letter, simulating a typewriter. The effect can even process HTML tags such as <B>
and <FONT>.
At the heart of this effect are
the use of these components:
-
innerHTML
property
-
setTimeout()
function
innerHTML
innerHTML is a property of an element that can be used to change the content of
the element. For example, this piece of code creates a DIV element
named "myDiv":
<DIV ID="myDiv"><B> This is the content of
myDiv </B></DIV> |
We can see what the innerHTML of
the "myDiv" element
looks like:
javascript:alert(document.getElementById("myDiv").innerHTML);
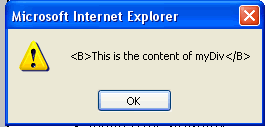
Notice that the innerHTML content not only contains the
text, but also the HTML tags within the element (the <B> and
</B>).
You can assign new values to the innerHTML.
For example, suppose your html contains:
<DIV ID="swappable">Good morning</DIV>
|
To change the text "Good morning"
to to "Good evening," you can do it like this:
<SCRIPT LANGUAGE="JavaScript">
<--
document.getElementById("swappable").innerHTML="Good evening";
//-->
</SCRIPT>
|
You can even add HTML
tags into the innerHTML. Below, I made the new text larger by
making it a header <H2>:
<SCRIPT LANGUAGE="JavaScript">
<!--
document.getElementById("swappable").innerHTML="<H2>Good evening</H2>";
//-->
</SCRIPT>
|
Note: innerHTML is not
part of W3C DOM standard and might be depreceated in the future.
An example of doing the same thing as above in W3C DOM is like below:
document.getElementById("swappable").replaceChild(
document.createTextNode("Good evening"),
document.getElementById("swappable").childNodes[0]);
|
That piece of code will place the text "Good evening" as
the first child element of the DIV element. To include the html
tags, such as <B>, you'll need to create another node and
make the text a child of that node. Obviously, much more effort will be
required.
|
setTimeout
This is a JavaScript method (function) that causes an
expression (which could be a piece of code or a function call) to be executed after a specified
delay. The format
is as follows:
setTimeout(code,
numberOfMilliseconds), where:
If you're not familiar with setTimeout,
you can read more about it here.
The typing effect is accomplished by
repeatedly changing the innerHTML content of an element (eq: a
DIV). The repetition and speed of the typing is
controlled by setTimeout.
Note: you could also use setInterval,
instead of setTimeout.
|
COMPLETE EXAMPLE
|
<html>
<head>
<SCRIPT LANGUAGE="JavaScript">
<!--
var text="content of text here";
var delay=50;
var currentChar=1;
var destination="[not defined]";
function type()
{
if (document.getElementById)
{
var dest=document.getElementById(destination);
if (dest)
{
dest.innerHTML=text.substr(0, currentChar);
currentChar++
if (currentChar>text.length)
{
currentChar=1;
setTimeout("type()", 5000);
}
else
{
setTimeout("type()", delay);
}
}
}
}
function startTyping(textParam, delayParam, destinationParam)
{
text=textParam;
delay=delayParam;
currentChar=1;
destination=destinationParam;
type();
}
//-->
</SCRIPT>
<title>JavaScript Typing Effect</title>
</head>
<body>
<DIV ID="textDestination">...</DIV>
</body>
<SCRIPT LANGUAGE="JavaScrip"t>
<!--
startTyping(text, 50, "textDestination");
//-->
</html>
|
Click here to open the example above
Click here to open another example
Let's take a look at the JavaScript
code first.
var text="Content of text here";
var delay=50;
var currentChar=1;
var destination="[not defined]";
|
The 4 variables on the top portion of the code are
global variables. The first one contains the text that will be
displayed. The second one is the delay (in milliseconds) between the
typing. And the third variable is a counter that will store the index of
the next characters to be typed. So, for example, if the screen
shows "Co", then index will be 2 because we have shown 2
characters. The last variable holds the name of the element where
the text will be typed into. These
variables can be changed by the function startTyping. When
you call this function, it also signals that the typing should start and
the function type() is called.
function startTyping(textParam, delayParam, destinationParam)
{
text=textParam;
delay=delayParam;
currentChar=1;
destination=destinationParam;
type();
}
|
Here's what type() looks like:
function type()
{
if (document.getElementById)
{
var dest=document.getElementById(destination);
if (dest)
{
dest.innerHTML=text.substr(0, currentChar);
//dest.innerHTML+=text[currentChar-1];
currentChar++
if (currentChar>text.length)
{
currentChar=1;
setTimeout("type()", 5000);
}
else
{
setTimeout("type()", delay);
}
}
}
}
|
This function is the code that puts the
content of the text into the innerHTML of the destination element. If first gets the element using getElementById.
It then checks if the destination element is valid (if may not be valid if
the browser does not support getElementById; if you have
forgotten to create the element on the html page; or if you mistyped the ID
of the element).
Let's look at some of the lines in more details:
dest.innerHTML=text.substr(0, currentChar);
|
substr(startIndex, endIndex) is a built-in
JavaScript function that returns a sub-string of a string. So, that
line extract a portion of the string (returns a sub-string of the string),
then assigns the sub-string to the innerHTML. This is how we chop the text so that it shows character by
character:
As an exercise, you could have used a more efficient method
by just adding the next character at every cycle, like this:
dest.innerHTML+=text[currentChar-1];
// Hint: need to clear the content at the beginning
Let's move on.
currentChar++
if (currentChar>text.length)
{
currentChar=1;
setTimeout("type()", 5000);
}
else
{
setTimeout("type()", delay);
}
|
Here, we increment the counter
(index of the next character to be shown) and check if the text has been fully
displayed. If the text has been fully displayed, it waits for 5000
milliseconds before starting over. You can change that number to something
else; or remove this line altogether to not repeat the typing sequence. If
the text has not been fully displayed, it calls setTimeout so that type()
will be called again after the specified delay.
Following the script is the html code:
<title>JavaScript Typing Effect</title>
</head>
<body>
<DIV ID="textDestination">...</DIV>
</body>
<SCRIPT LANGUAGE="JavaScrip"t>
<!--
startTyping(text, 50, "textDestination");
//-->
|
This is a very simple html example.
It simply creates a DIV
element with ID="textDestination". This element
is where the text will be typed into. At the end of
the html, I called startTyping, passing "textDestination"
as the destination for the typing effect. This call initiates the
typing sequence.
|
COMMENTS
|
-
To handle browsers
that does not support innerHTML, I suggest you write an alternate
version of the page. You can test the browser version and direct
user to another page immediately if the browser is not one of the
known working browsers.
-
If you want to be
DOM compliant, you can replace:
dest.innerHTML=text.substr(0, currentChar);
with
var textNode=document.createTextNode(text.substr(0, currentChar));
dest.replaceChild(textNode, dest.childNodes[0]);
However, the text
will be displayed as it is (ie: html tags will be displayed as
if they're text).
-
You can set the
width and height of a DIV element to make sure that there's
enough space for the text. However, you can't never
be sure what kind of font the user is using.
-
You can change
the innerHTML of a table cell, or a button, and many other
html elements. You must set an ID for
the element so that you can refer to it using getElementById().
-
You can change
the horizontal alignment the element to "CENTER" to make
the text appear from the center.
-
Using a table
cell, you can change the vertical alignment of the element to
"MIDDLE" or "BOTTOM" to make the text appear
from the middle or scroll up from the bottom. You can also use
similar method in DIV to accomplish this.
|
<< MORE TUTORIALS >>
(C)2003 F. Permadi
permadi@permadi.com
|
|